Summary
The use of ARIA roles, states and properties is necessary to ensure that custom controls are accessible to users of assistive technologies.
Techniques
-
Enable interaction using roles, states and properties. [[WCAG-4.1.2]]
-
Ensure ARIA roles, states and properties are valid on their specified elements. [[WCAG-4.1.1]]
-
Ensure all parent/child role requirements are satisfied. [[WCAG-4.1.1]]
-
Do not trigger events when a control recieves focus. [[WCAG-3.2.1]]
-
Do trigger unexpected events when a user inputs values or changes settings. [[WCAG-3.2.1]]
Example
The img
element has the role button
which will identify it as a button
and not an image to assistive technologies.
<img src="controls/start.png"
id="start"
role="button"
tabindex="0"
alt="Start"/>
The following simple example shows some of the considerations that must be paid attention to when creating a custom control: setting and unsetting callback functions, enabling and disabling the control, changing the visual appearance of the buttons. To make this image work with keyboards, the user's keypresses must also be monitored, and actions turned on and off.
<img src="controls/play.png"
id="start"
alt="Start"
role="button"
aria-disabled="false"
tabindex="0"
onclick="controlPlayback('start')"/>
<img src="controls/stop.png"
id="stop"
alt="Stop"
role="button"
aria-disabled="true"
tabindex="0"/>
<script>
<![CDATA[
function controlPlayback(action) {
var isStart = (action == 'start') ? true : false;
var start_image = isStart ?
'controls/play-disabled.png' :
'controls/play.png';
var stop_image = isStart ?
'controls/stop.png' :
'controls/stop-disabled.png';
var start = document.getElementById('start');
start.setAttribute('aria-disabled',
!start.getAttribute('aria-disabled'));
start.setAttribute('disabled',
!start.getAttribute('disabled'));
start.onclick = isStart ?
null :
function () { controlPlayback('start'); };
start.setAttribute('src', start_image);
var stop = document.getElementById('stop');
stop.setAttribute('aria-disabled',
!stop.getAttribute('aria-disabled'));
stop.setAttribute('disabled',
!stop.getAttribute('disabled'));
stop.onclick = isStart ?
function () { controlPlayback('stop'); } :
null;
stop.setAttribute('src', stop_image);
if (isStart) {
start.removeAttribute('onkeypress');
stop.setAttribute('onkeypress', "if (event.keyCode==32||event.keyCode==13) { controlPlayback('stop'); }");
}
else {
stop.removeAttribute('onkeypress');
start.setAttribute('onkeypress', "if (event.keyCode==32||event.keyCode==13) { controlPlayback('start'); }");
}
alert(isStart ? 'Playback started!' : 'Playback stopped!');
}
]]>
</script>
The following buttons show this code in action:
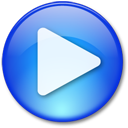
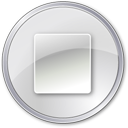
Explanation
Although HTML provides a number of native controls (e.g., input
, button
,
progress
), developers often have to create their own equivalents to avoid the
styling limitations or introduce specialized functionality.
The creation of custom controls can cause seriously accessibility issues, however, as the reliance on JavaScript to make the functionality work does not translate to assistive technologies by default. For one, when non-interactive elements like spans of text and images are made dynamic, assistive technologies will not be aware that the user can activate them. As well, as actions occur, the changes to the content are not reflected in the accssibility tree (i.e., the new content will not be available).
These scripting accessibility problems plagued early development on the web, but the introduction of ARIA roles, states and properties has helped mitigate many of the problems. Adding these attributes to custom controls makes assistive technologies of their presence and allows information about the current state of the control to flow through to users.
The following list describes the purpose of each of these types of attributes.
- Roles
-
The ARIA
role
attribute is used to describe the function of a custom control (e.g., to indicate that an image functions like a button).Custom controls require a role in order to inform assistive technologies about their purpose. This allows the assistive technology to present the element to users according to its expected use.
Refer to the ARIA specification for the current list of supported roles.
- States
-
State attributes identify the current status of a control: whether it is currently checked or not, whether it is hidden, etc.
State attributes must be maintained by script as the user interacts with the control/content, otherwise the user may get locked out of functionality (e.g., unable to reduce the volume, unable to make important content visible, etc.).
Refer to the ARIA specification for the current list of supported states.
- Properties
-
Property attributes identify meta information about a control, such as the minimum or maximum value it allows or its place within a group.
Unlike states, properties are typically only set once when the content loads.
Refer to the ARIA specification for the current list of supported properties.
There is more to making custom controls accessible than just assuring that the correct roles, states and properties are applied, of course. Authors also need to make sure that they do not trigger unexpected changes in context, for example, when focus or input is received by the control.